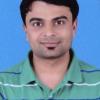
Setting up a database connection in Selenium and Cucumber involves a few key steps:
Database Driver and Dependencies:
You need to include the appropriate database driver or library in your project. For example, if you're using MySQL, you would typically use the MySQL Connector/J library.
Database Connection Details:
You'll need the database connection details, including the URL, username, and password.
Database Configuration:
Create a configuration file or store these connection details in a secure manner. You can use properties files, YAML files, or any configuration mechanism that suits your project.
Database Connection Class:
Write a class to manage the database connection. This class should load the driver, establish a connection, and provide methods for executing SQL queries.
In your Cucumber step definitions, you can use this DatabaseConnection class to interact with the database. For example, to execute SQL queries and validate data. Remember to handle exceptions and error scenarios properly in your code and make sure to close the database connection when you're done with it, typically in an @After method in your Cucumber setup.
Additionally, it's a good practice to externalize sensitive information like database credentials to configuration files and use best practices for handling secure data, such as encryption or environment variables.
- E.g
- import java.sql.Connection;
- import java.sql.DriverManager;
- import java.sql.SQLException;
- public class DatabaseConnection {
- private Connection connection;
- public DatabaseConnection() {
- try {
- // Load the database driver
- Class.forName("com.mysql.cj.jdbc.Driver");
- // Set up the database connection
- String url = "jdbc:mysql://localhost:3306/your_database_name";
- String username = "your_username";
- String password = "your_password";
- connection = DriverManager.getConnection(url, username, password);
- } catch (ClassNotFoundException | SQLException e) {
- e.printStackTrace();
- }
- }
- public Connection getConnection() {
- return connection;
- }
- public void closeConnection() {
- if (connection != null) {
- try {
- connection.close();
- } catch (SQLException e) {
- e.printStackTrace();
- }
- }
- }
- }